REST API's in Go
Creating simple REST end points in Go
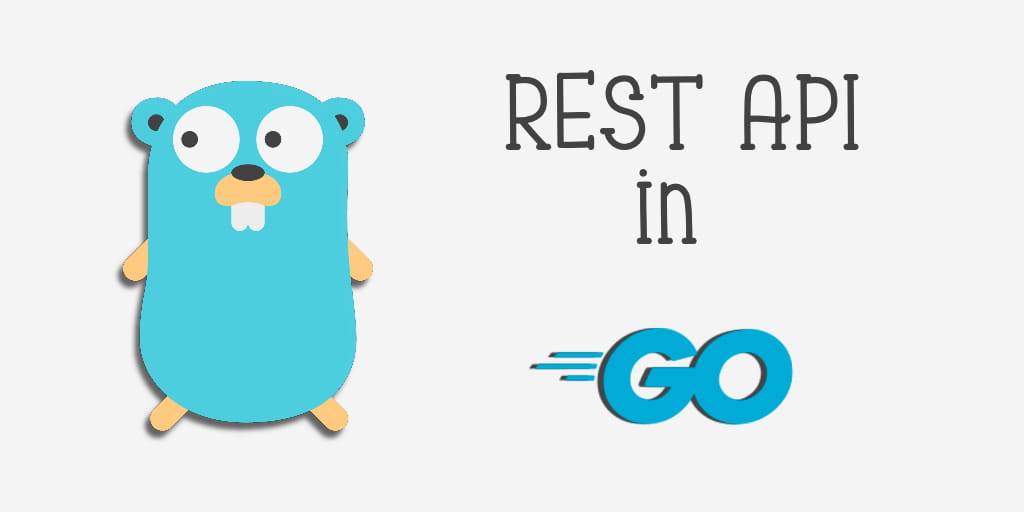
Simple reliable REST end point in open source programming language Go.
Install Go from https://golang.org/doc/install
URL to the working Go REST API Project : https://github.com/velusgautam/go-backend
First create the folder in for the project eg: go-backend
The main.go is the file master file. So we need Go to listen to a port (eg: 2001) for the requests and call respective functions based on the route path.
So to achieve that I created below folder structure. routes folder for routing, models for all the interfaces, handlers for action handling
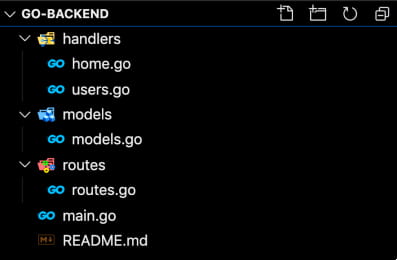
The folder names are important in Go.
Functions with capital names become Public. Also properties in struct should be Capital, keep a note on those
main.go
package main
import (
"fmt"
"go-backend/routes"
"log"
"net/http"
)
// handleRequest will call respective routes in HandleFunc
func handleRequest() {
for _, v := range routes.GetRoutes() {
http.HandleFunc(v.Route, v.Handler)
}
fmt.Println("Serving from : http://localhost:2001")
log.Fatal(http.ListenAndServe(":2001", nil))
}
func main() {
handleRequest()
}
Here we are looping through the routes and attaching respective handlers for the routes slice.
Listening to PORT:2001 in http.ListenAndServe
routes/routes.go
package routes
import (
"go-backend/handlers"
"go-backend/models"
)
type Route = models.Route
func GetRoutes() []Route {
var routes []Route
routes = append(routes, Route{Route: "/", Handler: handlers.Home})
routes = append(routes, Route{Route: "/count", Handler: handlers.GetUserCount})
routes = append(routes, Route{Route: "/users", Handler: handlers.GetUsers})
return routes
}
models/model.go
package models
import "net/http"
type User struct {
Name string `json:"name"`
Email string `json:"email"`
}
type UserCount struct {
Count int `json:"count"`
}
type Route struct {
Route string
Handler func(http.ResponseWriter, *http.Request)
}
handlers/home.go
package handlers
import (
"fmt"
"net/http"
)
// Home handler give the default page
func Home(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Welcome to Go REST SERVICE")
}
A home handler to show welcome page.
handlers/users.go
package handlers
import (
"encoding/json"
"go-backend/models"
"net/http"
)
// User model from models
type User = models.User
// UserCount model from models
type UserCount = models.UserCount
var users []User
// GetUsers handler to fetch all users
func GetUsers(w http.ResponseWriter, r *http.Request) {
users = append(users, User{Name: "John Doe", Email: "johndoe@gmail.com"})
users = append(users, User{Name: "Kim Jane", Email: "kimjane@gmail.com"})
users = append(users, User{Name: "Philip Mathew", Email: "pmathew@gmail.com"})
// setting content-type
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(users)
return
}
// GetUserCount handler to fetch the users count
func GetUserCount(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "application/json")
count := UserCount{Count: len(users)}
json.NewEncoder(w).Encode(count)
return
}
Public function to handle a list of users. path: /users
Public function to handle count of users. path: /count
Run the Service 🏃🏽♂️
go run main.go
The service will be listening to http://localhost:2001
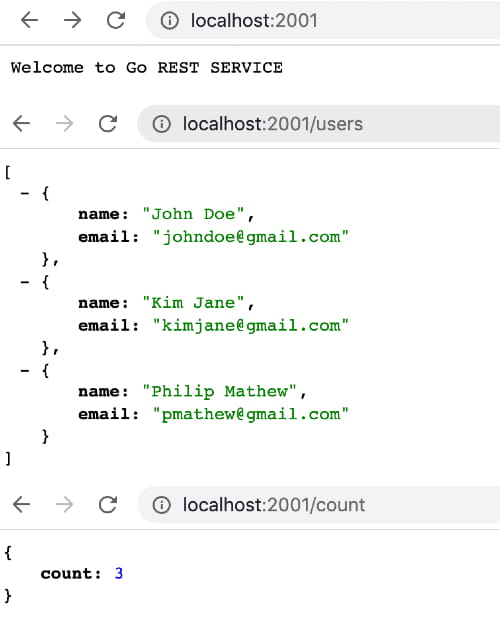