Date validation in javascript including leap year and invalid dates
Checking if the entered date is a valid date in client side and inform customer to create better UX experience. Checking invalid dates in leap years as well.
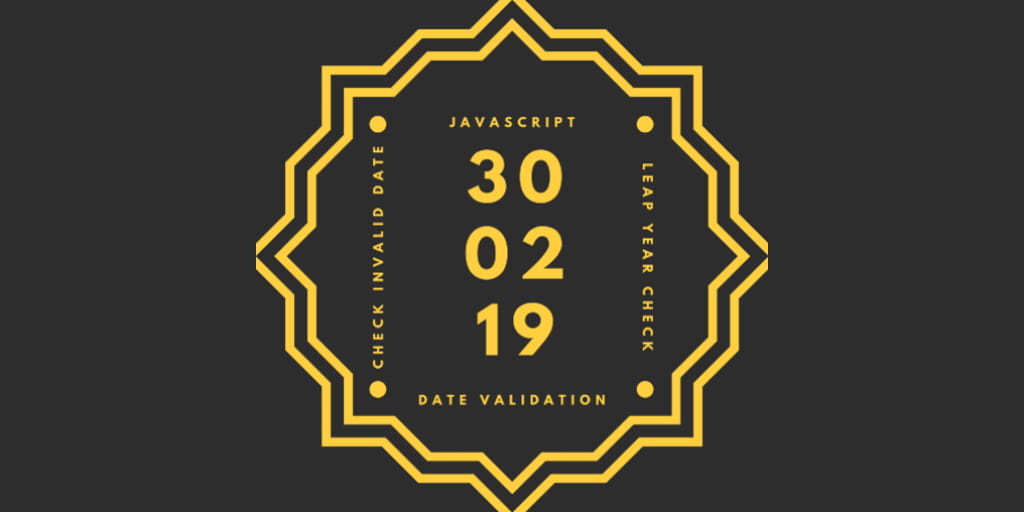
I was assigned a task recently to validate the date in the customer registration. There are a plenty of libraries like moment, luxon can help you achieve this. But for that simple validation I don't want to include a big package to my project. So I have written a small snippet that can do the job for you.
/**
* Checking if the input string date is valid or not
* @param dateInput {string} ["29-02-2000"]
* @returns {boolean}
*/
const validateDate = dateInput => {
if(dateInput.length < 10){
// invalid date if dateInput length is less than 10
return false;
}
// splitter will split the date and get the date seperator eg: -
const splitter = dateInput.replace(/[0-9]/g, '')[0];
// using splitter will get the parts of the date
const parts = dateInput.split(splitter);
// since year can be in front for yyyy-mm-dd and in last for dd-mm-yyyy taking care of that logic
const year = parts[0].length === 4 ? parts[0] : parts[2];
// month will be always in center
const month = parts[1];
// taking care of day for the different formats like yyyy-mm-dd or dd-mm-yyyy
const day = parts[0].length === 4 ? parts[2] : parts[0];
// creating date our of the year, month day
const date = new Date(year, +month - 1, day);
//validates leapyear and dates exceeding month limit
const isValidDate = Boolean(+date) && date.getDate() == day;
// isValid date is true if the date is valid else false
return isValidDate;
};
If you need to convert the string date and get the {year, month, day} the below snippet will help you.
/**
* Get Date Values in {year, month, day}
* @param dateInput {string} ["29-02-2000"]
* @returns {object}
*/
const getDateValues = dateInput => {
if(dateInput.length < 10){
// invalid date if dateInput length is less than 10
return null;
}
// splitter will split the date and get the date seperator eg: -
const splitter = dateInput.replace(/[0-9]/g, '')[0];
// using splitter will get the parts of the date
const parts = dateInput.split(splitter);
// since year can be in front for yyyy-mm-dd and in last for dd-mm-yyyy taking care of that logic
const year = parts[0].length === 4 ? parts[0] : parts[2];
// month will be always in center
const month = parts[1];
// taking care of day for the different formats like yyyy-mm-dd or dd-mm-yyyy
const day = parts[0].length === 4 ? parts[2] : parts[0];
// creating date our of the year, month day
const date = new Date(year, +month - 1, day);
// formatting the date to International datetime format to get the english standard
return {
year: new Intl.DateTimeFormat('en', { year: 'numeric' }).format(date),
month: new Intl.DateTimeFormat('en', { month: 'numeric' }).format(date),
day: new Intl.DateTimeFormat('en', { day: '2-digit' }).format(date)
}
}
You can do a date validation on top of the getDateValues also or can split the repeated code into different function for DRY principle.
So find if a date string is an invalid date or not in javascript.
Cheers✌🏻!!