Matrix Rotation in JavaScript
How to rotate a 2x2 matrix in javascript
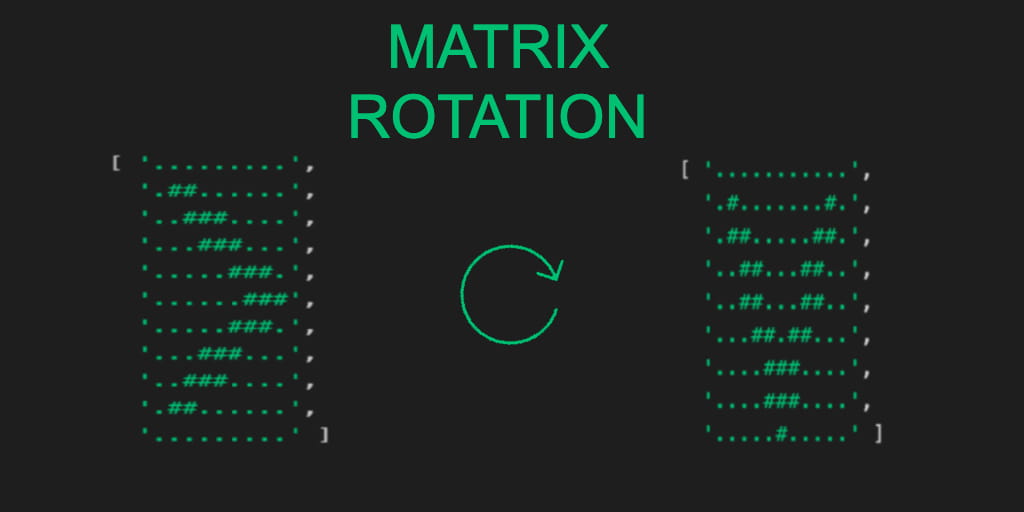
Rotating a matrix in clock wise direction using for loops.
Complexity Big O : n^2
This question was asked in live coding round of Frontend developer interviews in Flipkart, Walmartlabs
function rotateMatrix(matrix) {
result = [];
let len = matrix.length - 1;
for (let i = len; i >= 0; i--) {
var row = matrix[i].split('');
if (result.length === 0) {
result = row;
} else {
for (var j = 0; j < row.length; j++) {
result[j] = result[j] + row[j];
}
}
}
return result;
}
console.log(
rotateMatrix([
'.........',
'.##......',
'..###....',
'...###...',
'.....###.',
'......###',
'.....###.',
'...###...',
'..###....',
'.##......',
'.........',
])
);
console.log(rotateMatrix(["a","b","c","d"])) // ["dcba"]